Print to the Screen
Now, let’s try to print. No, not using a printer! Printing, or writing, characters on the screen.
So, what do I mean by characters? Not cartoon characters, or characters from a book of course. Instead, I’m talking about letters, numbers, and other symbols. Basically, anything you can type with the keyboard, and even more that aren’t on the keyboard (think of special letters that other languages use, for example).
Why is this important? When we write code, we usually need to tell the user what’s going on. This might be a message like ‘task has completed successfully’, or ‘Your tweet has been sent’. We may also need to write an error message, to tell the user that something went wrong.
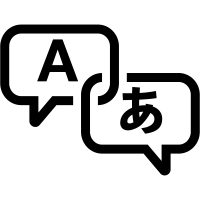
Let’s give it a go. Start by opening the Python shell, and typing in print (‘hello world’).
>>> print ('hello world') hello world
What happened? We gave the computer an instruction. We told it to print the words ‘hello world’ on the screen, which it did on the next line.
If this command didn’t work for you, check that you typed it exactly as I’ve shown you above.
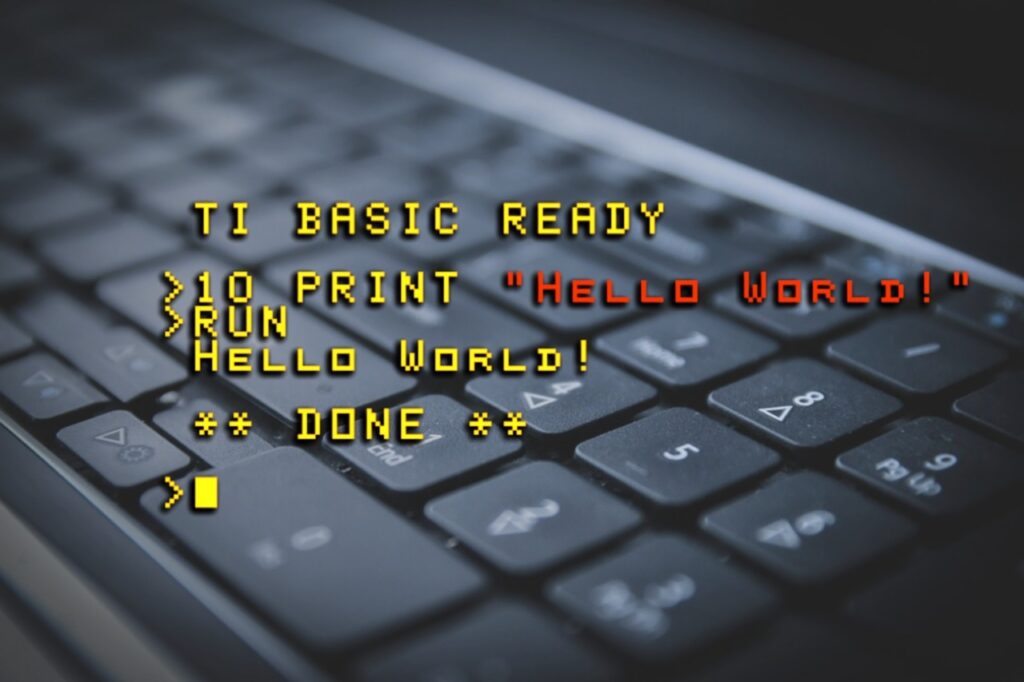
Did You Know?
The ‘hello, world’ program is a tradition in programming. It is often the first program a new student will write.
The first published example of this was in “The C Programming Language” from 1978
Functions
Let’s think about this example, and how it works. print is a function. A function is a block of code somewhere, often hidden from us.
Imagine that you’re at a restaurant. You look at the menu and decide what you want to order. You tell your waitress what you want. They go away, and eventually, they come back with your food.
This is great because you didn’t need to buy the ingredients, you didn’t need to prepare and cook the food. And when you’re done, you didn’t need to clean up.
Functions in Python are like this waitress. We don’t need to know every detail about how something is done, because someone else has already written that code for us.
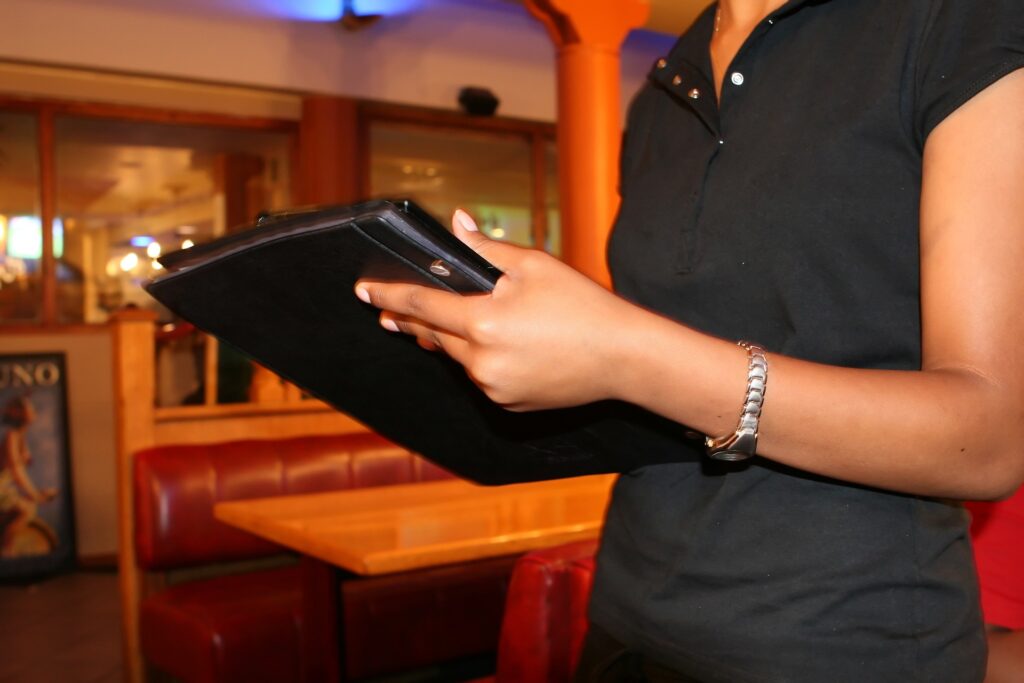
A ‘function’ is a reusable block of code
print is a great function because it saves us the bother of knowing how to print characters to the screen. We just tell Python to print, and it does the rest.
There are a lot of functions inside Python. Eventually, we’ll see how to build our own functions.
Functions are Reusable
The other reason that functions like print are so good, is because they are reusable. We can call the print function as often as we want. Functions mean we can write less code, and still have a good program.
Is THAT a Function?
We know functions when we see them because they use parenthesis. Parenthesis is the correct name for the opening and closing brackets.
Each function has a function name (like print), and then opening and closing parenthesis. Between the parenthesis, there may be one or more parameters. Parameters are extra information that we pass to the function.
Some functions need parameters. Some functions don’t have any parameters.
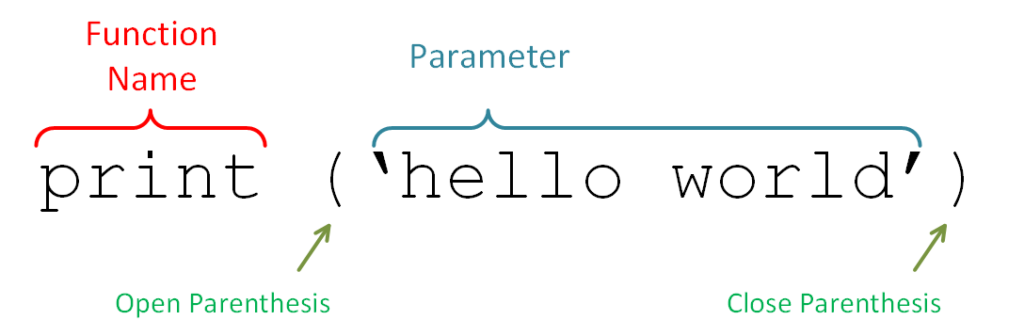
Printing Strings and Integers
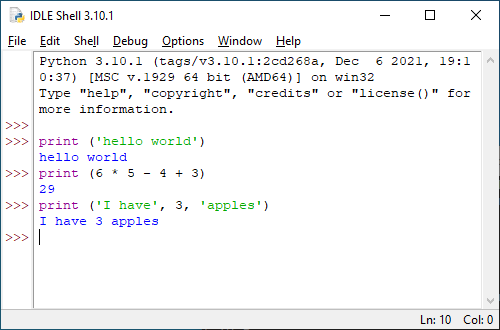
The print function can display different types of information. This includes strings and integers.
Next…
Next, we’re going to talk about variables. This is where the real programming begins!