Working with Variables
Let’s take a look now at some of the things we can do with variables. Get out the Python shell, and follow along!
Printing
First, let’s create the variable marbles, and assign the value of 10:
>>> marbles = 10
In the shell, we can simply write the variable name to print the contents:
>>> marbles
10
Or, we could do it the ‘proper’ way with the print function:
>>> print (marbles) 10
When we use print like this, we’re asking Python to print the contents of the marbles variable.
Math
If our variable contains a number, we can also add, subtract, multiply, and divide the contents. Try this:
>>> marbles = marbles + 5 >>> print (marbles) 15
The marbles variable originally contained the value of 10. Now we have added another 5, to make 15. The marbles = marbles + 5 part might look a bit confusing at first.
To understand it better, I suggest thinking of the part of the right-hand side of the assignment operator (that’s the equals symbol) first. You know that marbles (the variable) contains 10 (the value), and we’re adding 5 to that. The result is 15, which we assign back to marbles.
Once you’re comfortable with that, we can think of a simpler way to write it:
>>> marbles += 5 >>> print (marbles) >>> 20
This does the same thing the previous example did. It adds another 5 into marbles, to make 20. We can do the same thing with subtract, multiply, and divide:
>>> marbles -=4 >>> print (marbles) 16 >>> marbles *= 2 >>> print (marbles) 32 >>> marbles /= 4 >>> print (marbles) 8.0
Did you notice something interesting in this example? When we divided marbles by 4, the result wasn’t 8. It was 8.0
What does this mean? Do you remember when we discussed strings and integers? We talked about how they are different types of information. That is, they are different data types. Strings contain text, which integers are whole numbers.
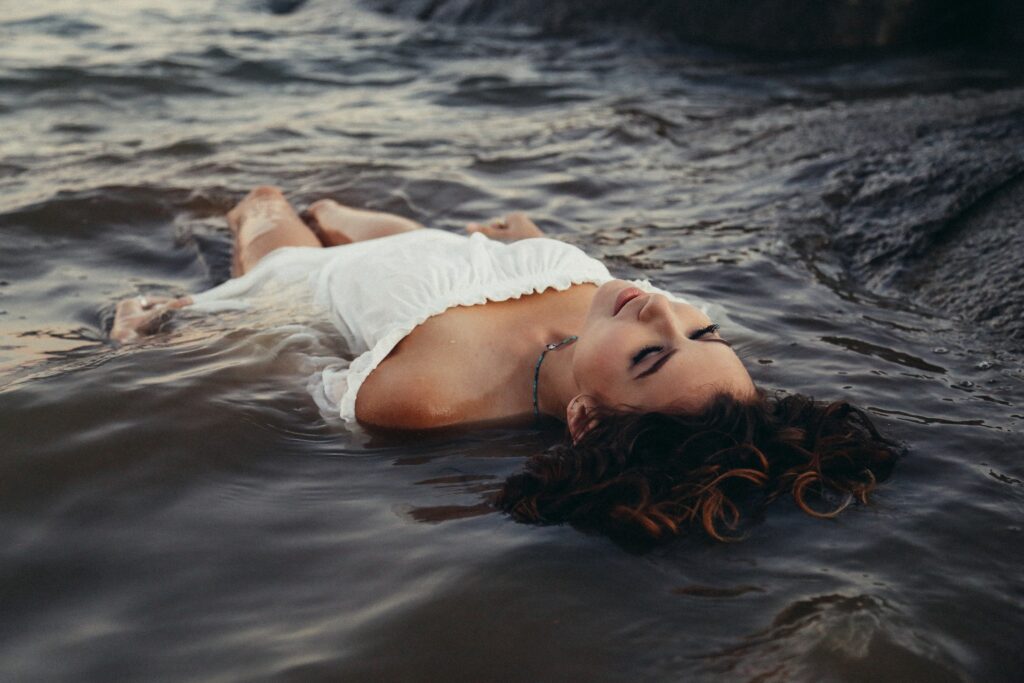
We have now seen a new data type called a float. This is short for floating-point number. There is a complicated explanation for these, but to simplify it, its numbers with a decimal point. These are numbers that may not be whole numbers.
When we take a whole number (and integer) and divide it by something, the result may not be a whole number anymore. Because of this, when we divide something, Python will convert the integer into a float.
Assignment
When we used marbles = 10, we assigned the value of 10 to the variable called marbles. We can also assign the value in one variable to another. Let me show you what I mean.
First, we’ll assign values to these two variables:
>>> marbles = 10 >>> lego = 55
We can add these variables together, and store the result in a different variable:
>>> toys = marbles + lego >>> print (toys) 65
We created a new variable called toys. We then took the value in marbles (10) and added it to the value in lego (55). We took the result (65) and placed it in the toys variable.
Strings
Numbers aren’t the only data types we can store in a variable. We can use strings as well. Try this one:
>>> password = 'SuperSecret!1' >>> print (password) SuperSecret!1
We created a new variable called password and put a string in it. We know this is a string because it is using quote marks.
Data Types
Earlier we spoke about data types again. We have now discussed three different data types:
- Strings – Text
- Integers – Whole numbers
- Floats – Numbers with a decimal point
How can we tell what data type is in a variable? Open up the Python shell, and I’ll show you!
First, let’s create these three variables. Each will contain a different data type:
>>> a = 'Hello World' >>> b = 42 >>> c = 3.8
There is a function in Python called type. This will tell us the variable’s data type:
>>> type (a) <class 'str'> >>> type (b) <class 'int'> >>> type (c) <class 'float'>
This shows us that the variable, a, has the type str (short for ‘string’). Variable b has the type int (short for ‘integer’). Variable c has the type float.