Python Practice – Converting Values
Let’s try out the skills we’ve covered so far with this practice session. You will need to try converting between different values using Python.
Try to work through the practice on your own, and come to your own solution. You will get the most benefit if you try to solve as much of this as you can on your own. This is not a test – You can go back to the examples we’ve already used, or use a search engine (Google, etc) if you want to.
If you need help, I have the solution down below.
The Challenge
You need to write a program to achieve these goals:
- Weight – Convert pounds to kilograms
- Weight – Convert kilograms to pounds
- Distance – Convert miles to kilometers
- Distance – Convert kilometres to miles
- Temperature – Convert Fahrenheit to Celsius
- Temperature – Convert Celsius to Fahrenheit
The answer should be printed to the screen. You may decide to put this into one big program or make a small program for each conversion. It’s really up to you.
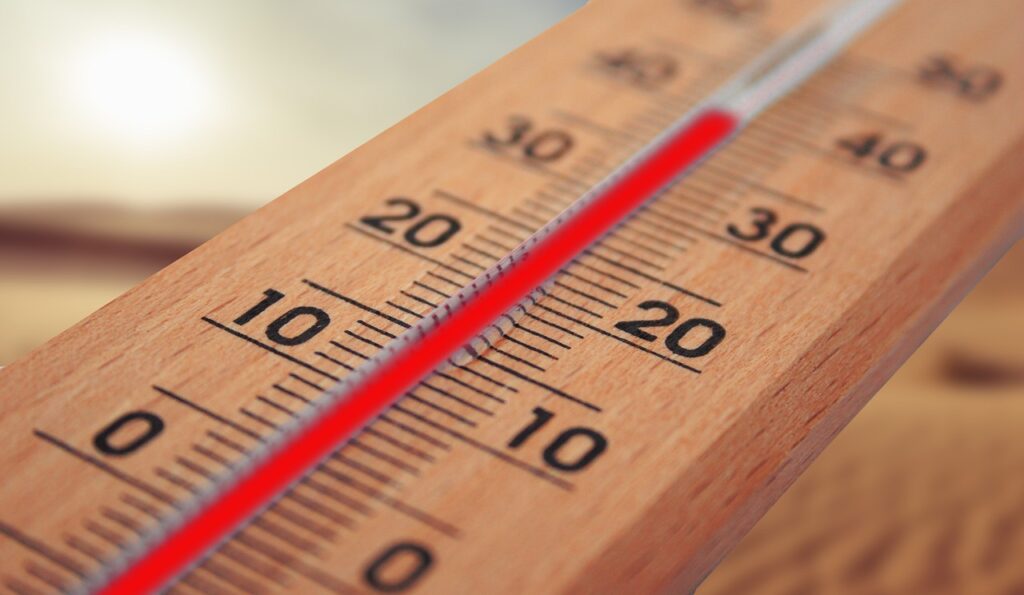
The Solutions
I hope you were able to work this puzzle out on your own. If not, that’s ok, the solutions are explained below.
Before writing any code, the first thing I would recommend is to learn how these conversions work. That is, use Google to see how to convert between pounds and kilograms, and so on. When you understand that, writing the code is easier.
Weight
A quick google search shows that to convert pounds to kilograms, we multiply the number of kilograms by 0.45
We can put this into code simply:
# Convert from pounds to kilograms pounds = 5 result = pounds * 0.45 print (pounds, 'pounds is', result, 'kilograms')
In the example above, we have a variable named pounds. We’ve set this to 5 pounds for now, but you can change it to whatever value you want.
We have a second variable called result. We multiply the number of pounds by 0.45, and store the answer in result.
Finally, we print the answer to the screen with the print function. We send print a list of four items in this case.
You may have noticed that there’s another way to convert pounds to kilograms. Rather than multiply the number of pounds by 0.45 (as above), we could have divided by 2.2
This shows that there’s often more than one way to approach a problem. Both ways come to the right answer.
To convert from kilograms to pounds, we can simply do the reverse. Rather than multiplying by 0.45, we can divide by 0.45:
# Convert from kilograms to pounds kilograms = 5 result = kilograms / 0.45 print (kilograms, 'kilograms is', result, 'pounds')
Distance
To convert miles to kilometers, multiply the number of miles by 1.609
# Convert from miles to kilometres miles = 5 result = miles * 1.609 print (miles, 'miles is', result, 'kilometres')
Converting kilometers to miles is simply the reverse. Divide the number of kilometers by 1.609:
# Convert from kilometres to miles kilometres = 5 result = kilometres / 1.609 print (kilometres, 'kilometres is', result, 'miles')
Temperature
Converting temperature is a little bit trickier. To convert Fahrenheit to celsius, we first need to subtract 32 from the temperature. Then we can divide the result by 9/5.
That sounds a little complicated, but for coding purposes, it’s really not too hard:
# Convert from fahrenheit to celsius fahrenheit = 100 result = (fahrenheit - 32) / (9/5) print (fahrenheit, 'degrees fahrenheit is', result, 'degrees celsius')
As you can see in the example above, we can do the entire calculation on a single line. We can also use brackets (parenthesis) in our math, to make sure the calculation happens correctly.
Converting the other way is just the reverse again. Multiply the temperature (in celsius) by 9/5, and then add 32:
# Convert from celsius to fahrenheit celsius = 100 result = celsius * (9/5) + 32 print (celsius, 'degrees celsius is', result, 'degrees fahrenheit')
And that’s the solution to all these conversions! I hope you understand how this works. If not, please leave constructive feedback below.
Next…
Next, we will start learning about how to get Python to make decisions