Boolean Data Type
Boolean is another data type that exists in most (if not all) programming languages. We’ve already talked about the integer data type, which is a number, and the string data type, which is text.
Boolean is a bit different. There are only two possible values. These are true and false. It is called a logical data type, as it is used with the computer’s ‘logic’ (the way it makes decisions).
As you can imagine, boolean is often used with expressions. That is, asking the computer a question, and getting a true or false answer. For example, asking Python if 1 > 0 results in a response of true (a boolean value).
Inside the guts of Python, the number zero represents false. Any other number represents true.
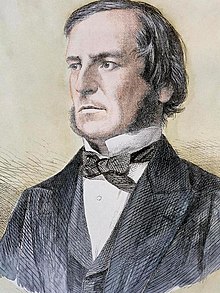
Did You Know?
The Boolean data type was named after English mathematician George Boole. He was one of the pioneers of mathematical logic.
bool() Function
A simple way to see boolean in action is to use the print function. In the example below, python will print False to the screen.
x = 5 y = 0 print (x == y)
Another option is to use the bool() function:
x = 5 y = 0 bool (x) bool (y)
In this example, Python returns True and False respectively. Can you see why? It’s because x is non-zero, which means true. And y is zero, which means false.
This makes it easy to see if a variable has a value at all. We can simply check if the variable is true.
Here is another example using expressions:
x = 5 y = 0 bool (x > y) bool (x == y)
This also returns True (because x is greater than y), and False (because x does not equal y).