Making Decisions with If and Else
To really get the value out of a programming language, we need to make it ‘think’, or make decisions. We can do this with an ‘if else’ block of code.
When we use ‘if‘, we’re asking Python a question. It will answer our question, but it will only answer true or false. It’s like playing the twenty-questions game with a computer.
Depending on the answer to that question, we can then ask Python to take some action (such as printing a message to the screen).
Think of this simple example. A nurse may check a patient’s temperature. If the temperature is too high, they will go and get a doctor.
Python, as well as other programming languages, will take this more literally. It will ‘think’ like this:
“The temperature is 38.5 degrees Celsius, and fever is 38 degrees Celsius. The temperature is higher than fever, so I will print a warning message on the screen”
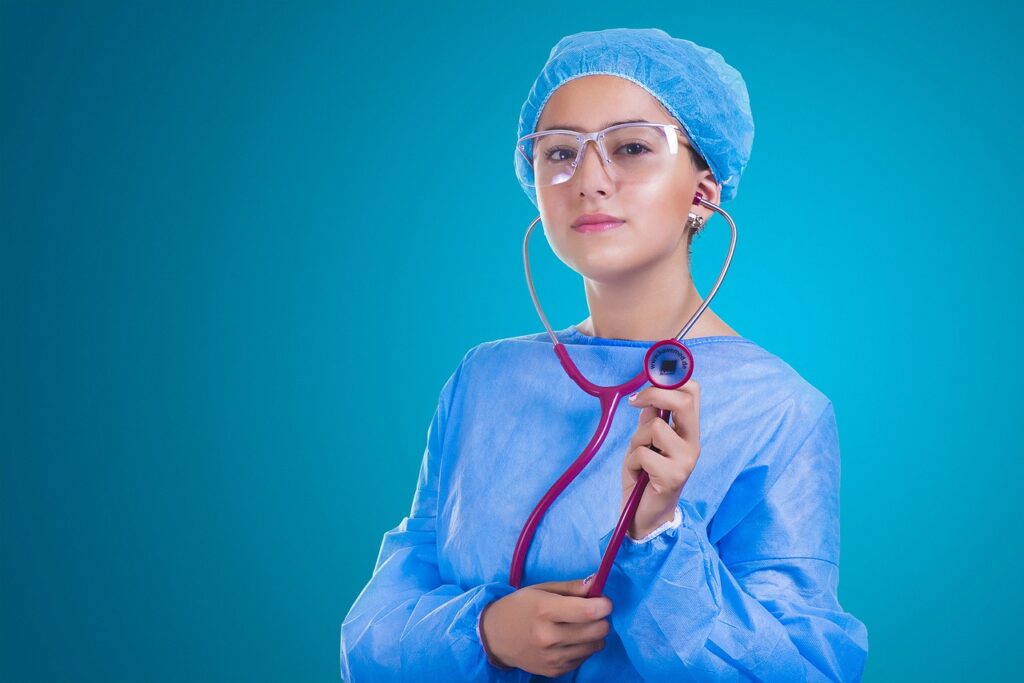
Asking Questions and Getting Answers
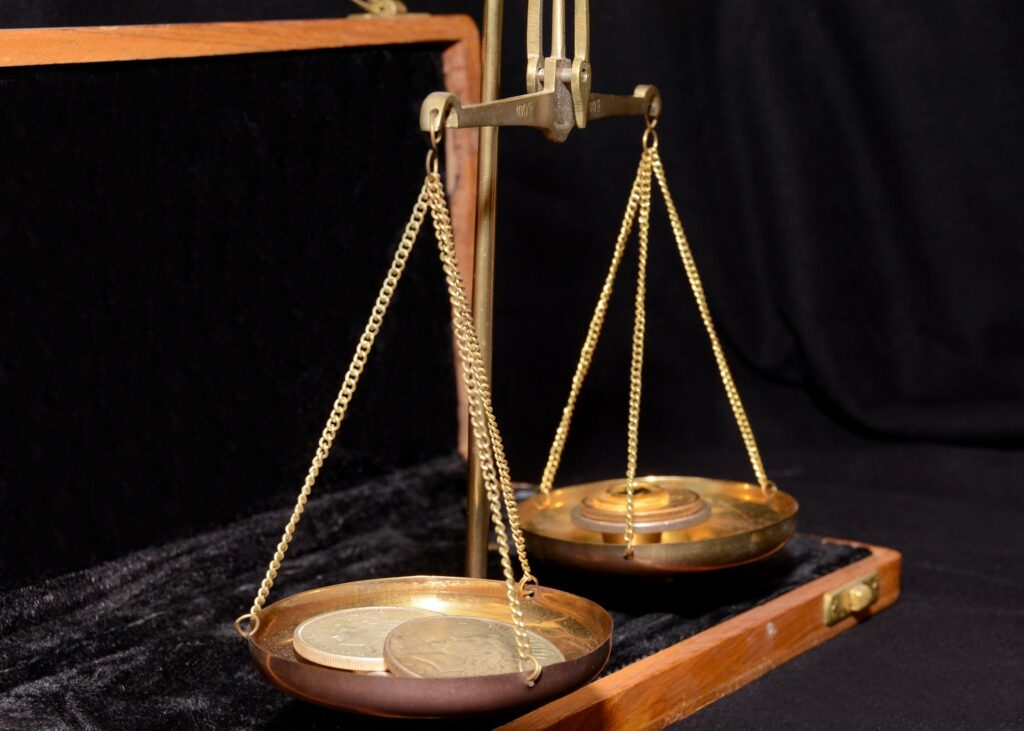
Comparing Values
We ask questions and Python answers. But the way Python handles questions is very different to how people do.
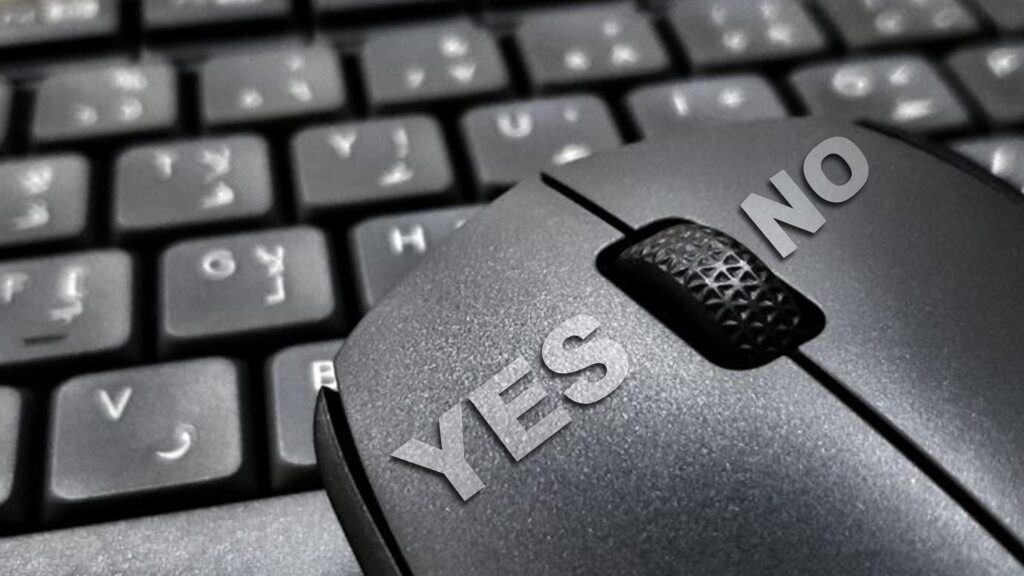
Boolean – True and False
Python answers all questions with a yes or no. Or really, true or false. This is known as Boolean Logic.
Using ‘If’
Now that we can ask Python questions, we can get it to make its own decisions. The most common way to do this is with the ‘if‘ statement. The logic behind this is:
Hey Python, IF this is true, THEN do this…
Maybe we should look at an example… Try this one out:
temperature = 38.1 fever = 38 if (temperature >= fever): print ('This patient's temperature is', temperature) print ('This is a fever, call the doctor!')
Let me explain what’s happening here.
First, we’ve set two variables. The first one is the patient’s temperature, in Celsius (feel free to change to Fahrenheit if you wish). The second one is the temperature of a fever.
Next, we’ve got an ‘if‘ block. This starts with us asking Python if temperature is greater than or equal to fever. The part in the parenthesis (brackets) is the expression. In this case, the answer is true. Also, notice that this line of code has a colon symbol at the end.
Because the answer is true, Python will run the code in the ‘if‘ block. In this example, it prints two messages to the screen. If the answer was false, it would ignore this code.
So an ‘if‘ block is where we ask Python a question, using an expression. Python will answer with true or false. If the answer is true, Python runs the code after the colon symbol.
Indenting
You might have noticed something interesting. Inside the ‘if‘ block, the code (where we use print) starts with four spaces. This is called indenting.
This is extremely important in Python. We’re telling Python that these lines of code are part of the ‘if‘ block. It’s also a very tidy way of writing our code.
This means that under the ‘if‘ line, we can have one or many lines of code. They’re all run in order if the expression is true.
Else
Let’s change our example a bit:
temperature = 37.5 fever = 38 if (temperature >= fever): print ('This patient's temperature is', temperature) print ('This is a fever, call the doctor!')
What happens now? Well, temperature (37.5) is less than fever (38). This means that the expression (temperature >= fever) is false. Because this is false, the lines of code in the ‘if‘ block are not run. In fact, this is the end of our code, so nothing else happens at all!
What if we want to print a message saying that this patient’s temperature is fine? We can do this, using ‘else‘:
temperature = 37.5 fever = 38 if (temperature >= fever): print ('This patient's temperature is', temperature) print ('This is a fever, call the doctor!') else: print ('This patient\'s temperature is fine')
In this example, we’ve extended the ‘if … else‘ block. The ‘else‘ statement tells Python what to do if the expression is false. Notice that ‘else‘ also ends with a colon, and the code under it is indented.
It is optional to pair an ‘else‘ with an ‘if‘. We won’t always need them, but they can be very useful from time to time.
Escape Characters
In the final line of the ‘if … else‘ block, I wrote patient\’s. Why is there a back-slash in there? Is that a mistake?
No! it’s not a mistake! This is an escape character. You know we wrap our text in quote symbols to print it to the screen? Well, what happens if we want to print a quote mark to the screen? Normally this would cause a problem.
That’s what the back-slash is for. It says “This next character is not special this time. Just print it to the screen please”
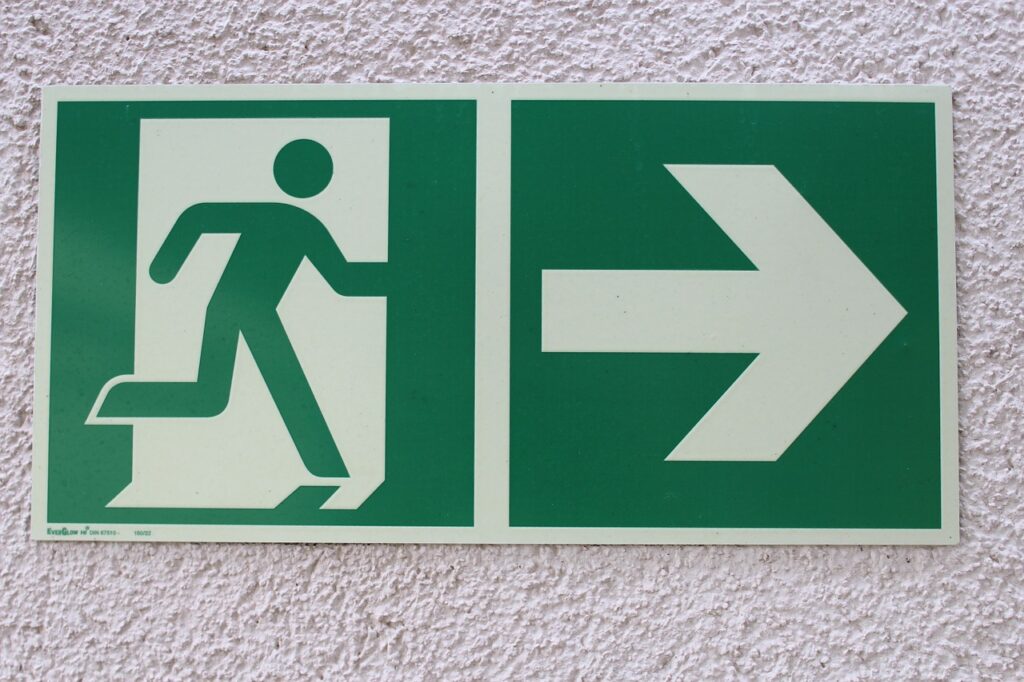
Else If
There’s still more we can do with an ‘if … else‘ block! What would happen if we also wanted to check if the patient’s temperature is too low? In this case, we can do something like this:
temperature = 35.8 fever = 38 chill = 36 if (temperature >= fever): print ('This patient's temperature is', temperature) print ('This is a fever, call the doctor!') elif (temperature <= chill): print ('This patient's temperature is', temperature) print ('This is too low, call the doctor!') else: print ('This patient\'s temperature is fine')
First, we’ve added a new variable called chill. This is when the body’s temperature is getting too low.
Second, we added an ‘elif‘ section. This is short for ‘else if‘. When the ‘if‘ expression is false, Python will look at the else if expression. When the ‘else if‘ expression is true, the code under it runs. If it’s false, Python moves on to the else section.
We can add as many ‘elif‘s’ as we want. The only rule is that they must be after the ‘if‘, and before the ‘else‘.
Try this yourself to get a bit of practice. Change the value of temperature, and see the effect it has on the program.
Next…
In the next article, we’re going to explore the list data type further.