Lists – Another Data Type
We’ve already used a few data types. We’ve especially used integers (whole numbers) and strings (text). We’ve even talked about boolean (true/false) and floats (numbers with decimal points).
We’ve also seen that we can pass a list of items to the print() function. We’re now going to dive deeper into this data type: The list.
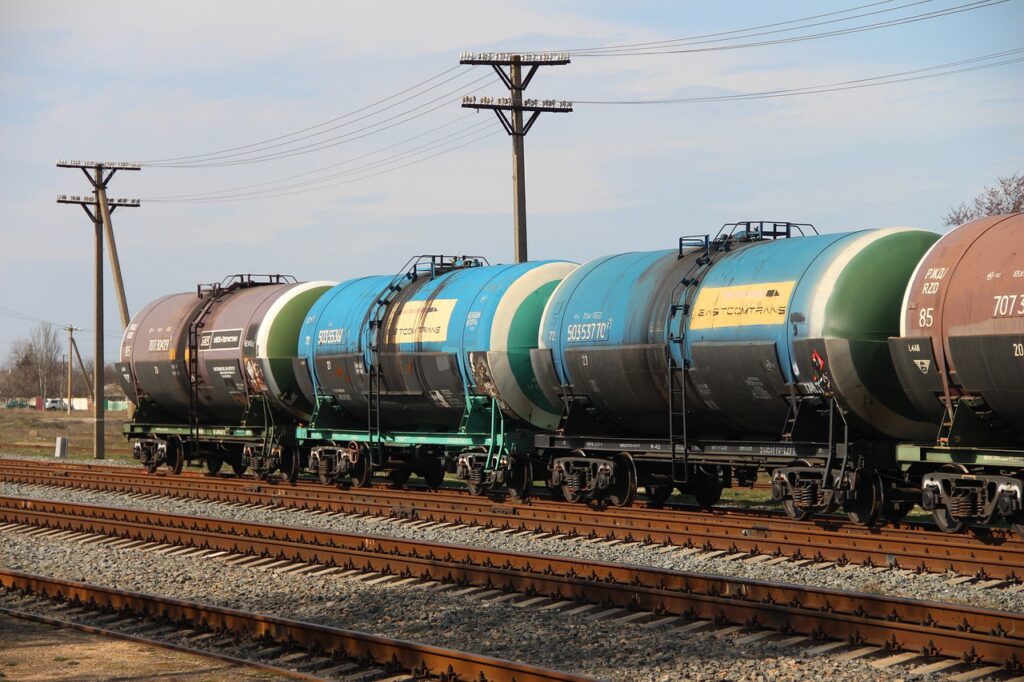
A list is a collection of items. These items can be different data types. For example, one item may be an integer, while another may be a string. A list can even contain other lists!
Think of a list as a line of train carriages. Each carriage contains something, but they can all hold something different.
A list is a line of items. Each item contains some data, but each item can hold different types of data.
Lists vs Arrays
Sometimes people will use the term array when they’re talking about a list. Arrays are also a data type, and it’s true that they share many things in common with lists.
As far as Python is concerned, arrays and lists are different things.
Arrays are a more advanced topic, so we won’t be talking about them any more here.
Creating a List
Just like all the other data types, we can store a list in a variable, using the assignment operator (the ‘equals’ symbol).
All we need to do is put our items inside square brackets. Each item is separated with a comma:
>>> mylist = [1, 2, 3, 4, 5] >>> print (mylist) [1, 2, 3, 4, 5]
Accessing Items
Each item in the list is called an element. Each element is numbered, so we can access it easily. This number is called the index.
The part that might be confusing, if you don’t already know this, is that computers start counting at zero. That means the first item in the list is element number 0. The second item is element number 1, and so on. 0 and 1 here, are each element’s index.
To see an item in a list, we can put the index in square brackets after the variable name. For example:
>>> mylist = [1, 2, 3, 4, 5] >>> # Show the first item (element #0) >>> print (mylist[0]) 1 >>> # Show the third item (element #2) >>> print (mylist[2]) 3
Always remember that computers start counting at zero.
Strings and Lists
‘Strings’ are a text data type. They contain a string of letters, numbers, or other characters.
It’s interesting to know that strings also act as a list. Each character in the string is an item in the list. Try this:
mystring = 'Hello' print (mystring[1])
Changing List Items
- we can also change items in a list
- for this, we just use the assignment operator (=)
- of course, refer to the element to change
- Doesn’t even have to be the same data type, we can throw a string in there
- use ‘+’ to concatenate lists
mylist[3] = 'Hello World' print (mylist)
The Things We Can Do!
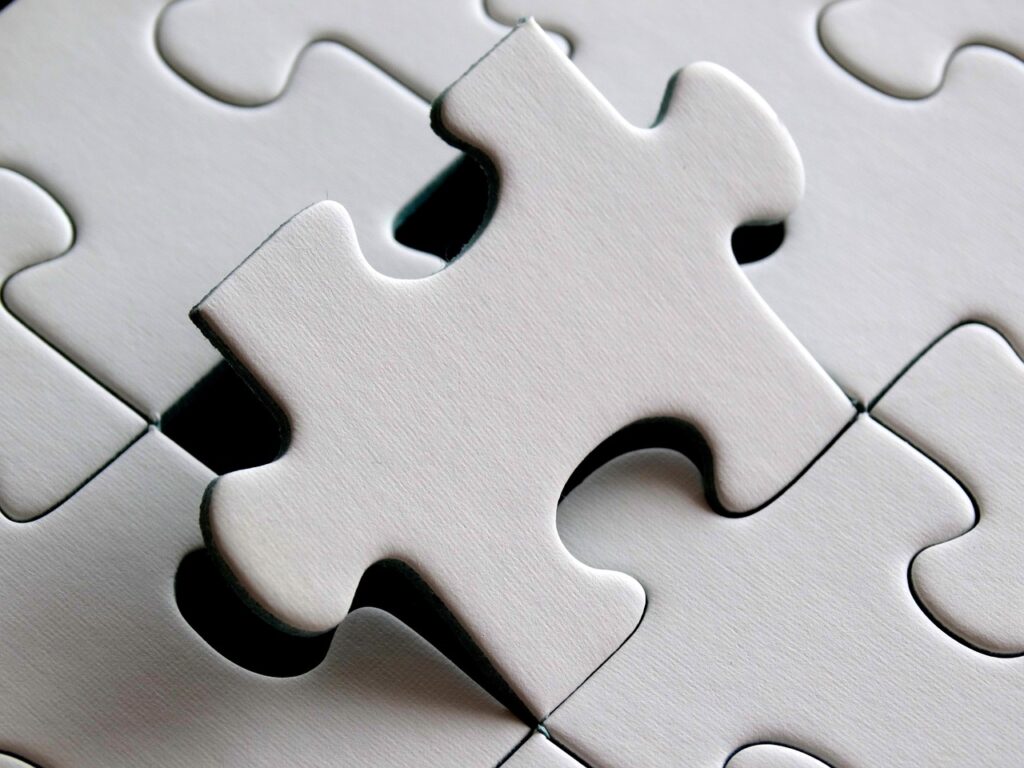
Fun with Lists
There’s a lot of ways we can use lists. Come and see just a few of the useful things we can do.
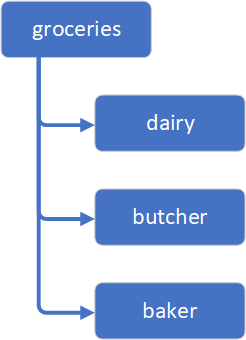
Lists of Lists
Lists can contain pretty much anything. That even includes other lists! This is another way that we can organize our information
Next…
Next up things get loopy… We’re going to see how (and why) to get Python to loop through code using ‘for’ and ‘while’ loops.