Looping Through Code
A large part of what computers do is handle repetitive tasks. That is, the boring stuff that loops over and over again. They’re also very good at it (and of course, they don’t get bored as we do).
Think of when a computer prints text onto the screen. It finds the next character to print, then prints it to the screen. It then moves on to the next character and repeats. It’s so fast you normally just see all the text at once, but in the background, it’s writing one letter at a time, just like we would.
Let’s think of another example. Say you have a variable, ‘x‘, and it is set to ‘1’. You would like to count from 1 to 5, and print each value to the screen.
You could write code that follows these steps:
- Set x to ‘1’
- print x
- Add 1 to x
- print x
- Add 1 to x
- print x
- Add 1 to x
- print x
- Add 1 to x
- print x
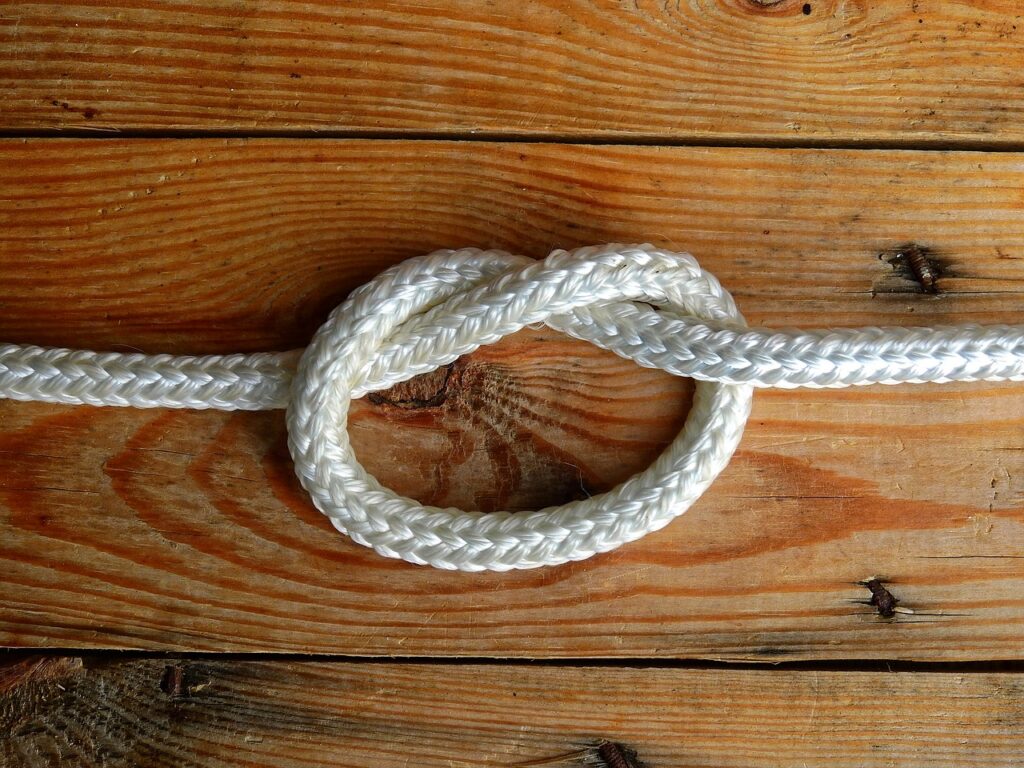
That would work. You would have the numbers 1 to 5 on the screen as you wanted. But it’s not a very good way to do it. What would happen if you wanted to count to 1000? Or even counting forever, until someone presses a button?
Can you see how much code you would need to write? A better way would be writing code that does this:
- Set x to ‘1’
- print x
- Add 1 to x
- Repeat steps 2 and 3 four more times
For Loops and While Loops
There are two different types of loops in Python. These are called ‘For‘ loops and ‘While‘ loops. They’re not unique to Python though, many programming languages will have them.
They both allow us to tell the computer to repeat tasks over and over again. While they’re similar in many ways, the way we use them is quite different.
For Loops
We use a ‘for’ loop when we (or the computer) know how many times the loop should run. This would make it a good choice for the counting to 5 example earlier.
This includes if we want to read items in a list. The computer knows how many items there are in the list. A ‘For’ loop can:
- Read the first item
- Do something with it
- Repeat until all items have been read
While Loops
A ‘While’ loop runs until it is told to stop. This makes it a good choice when we don’t know how many times we want the loop to run.
Think of a computer displaying a slideshow of pictures. It might use this logic:
- Display a new picture
- Wait five seconds
- If a user pressed a button, end the slide show
- If not, repeat back at step 1
Interrupting the Loop
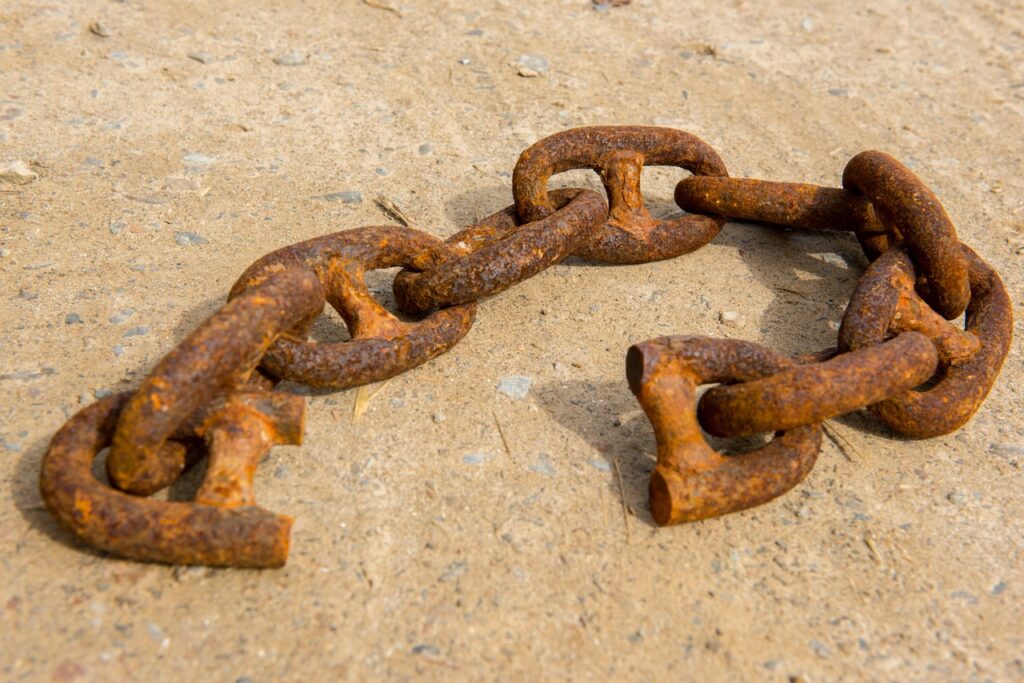
Usually, a loop will run a certain amount of times, or until a condition is met. But, there are times when we want to end a loop early. For example, if we find an error, or we find something that we’re searching for.
We can interrupt a loop by using the ‘Break‘ and ‘Continue‘ statements.
Next…
We’ll now look at how we can grow Python’s ability, and make our lives easier, by using ‘modules’ or ‘libraries’.