‘While’ Loops
A ‘While‘ loop will continue iterating until it’s told to stop. A While loop has an expression, much like an ‘if … else‘ block. While that expression evaluates to true, it will keep looping. That makes it quite possible for a loop to run forever, and never end!
Here is a simple example. Try running this code yourself, and see what happens.
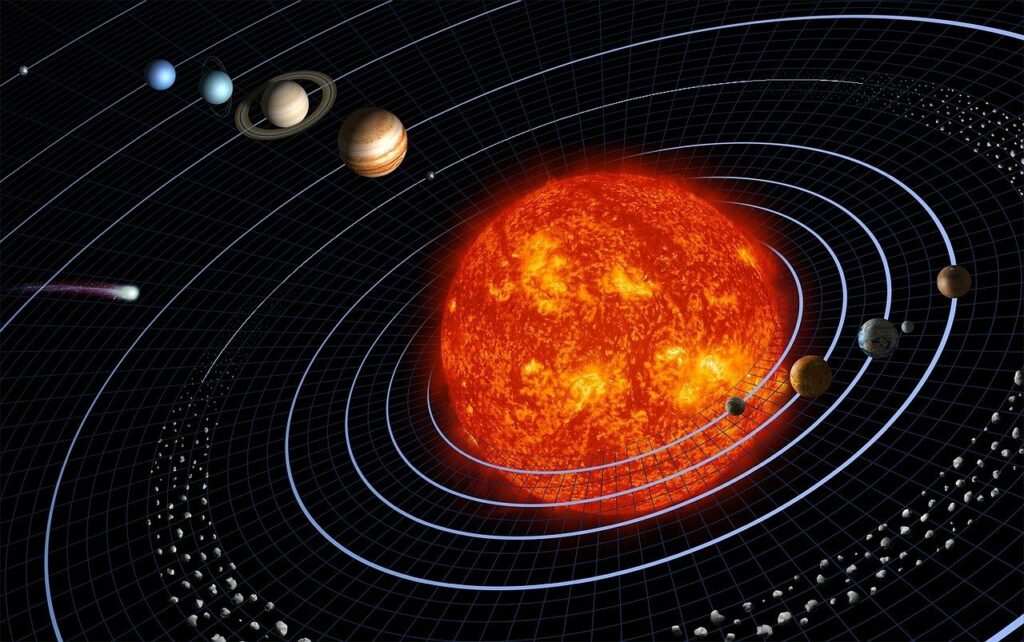
x = 0 while x < 6: print (x) x += 1
The While loop has the expression ‘x < 6‘. For the first iteration, x is zero, so this expression evaluates to true. Because the expression is true, the code inside the While loop will run.
This code will do two things. First, it will print the value of ‘x’. Then, it will add 1 to the value of x. Once that’s complete, the loop will run again.
It will continue looping (iterating) until the expression is false. This will happen when ‘x’ finally contains ‘6’.
Can you see what might go wrong if we don’t add 1 to ‘x’ in every loop? Try it and see!
Reading a String
Let’s look at another example. Try running this code:
sentence = 'Hello World' x = 0 while x < len(sentence): print (sentence[x]) x += 1
This while loop is a bit more advanced. The purpose of it is to take a string and print each character one at a time. The interesting part is in the expression, ‘x < len(sentence)‘.
The len() function gets the length of a string. That is the number of characters in the string. The ‘x’ variable is set (or initialized) to zero, which represents the first character of the string. ‘x’ is updated in every loop.
So, the expression is true as long as ‘x’ is less than the length of the sentence. Once ‘x’ moves beyond the last character in the sentence, the expression is false, and the looping stops.
Try testing this out by changing the string to something else. How might you print every second letter?