‘For’ Loops
When we use a ‘for‘ loop in Python (also known as a ‘For Each‘ loop in other languages), we give it a set of information to work with. This could be something like a range of numbers, or a list.
We also give the ‘for’ loop some code. This code runs for each item that we’ve given it.
This will be easier to see in this example. Please try this code out, and see the result.
for x in range (0, 5): print (x)
This is a very simple ‘for‘ loop. The first line creates the loop, and explains how it will work. There are two parts to this; The ‘for‘ part, and the ‘in‘ part.
Let’s start with the ‘in‘ part. This controls how many times this loop will run. In our example, we have given the loop a range of numbers (from 0 to 5), using the range() function.
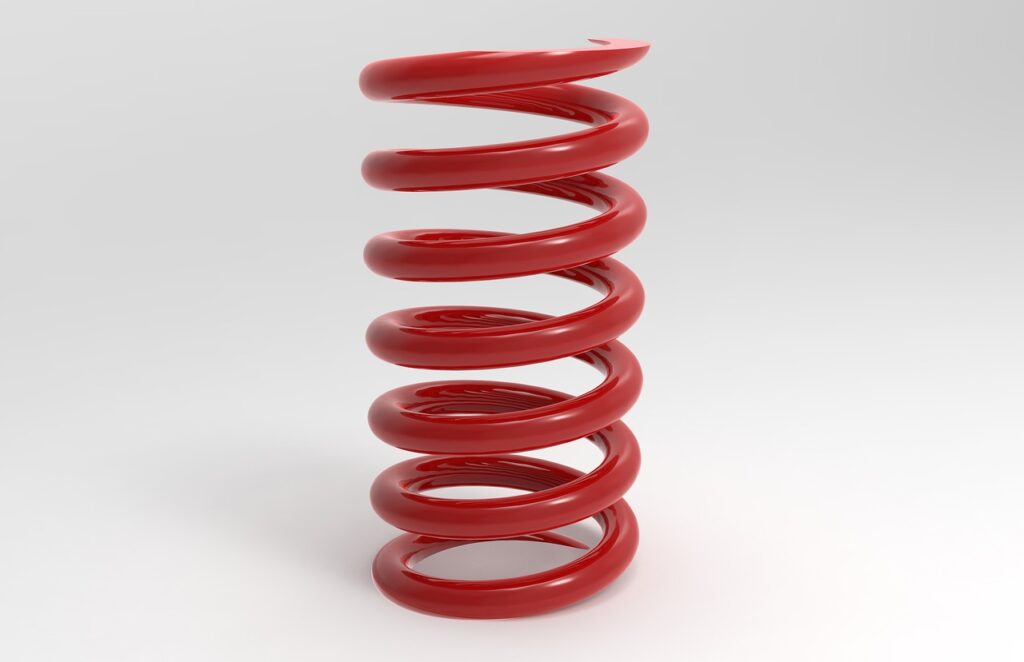
This means that the loop will run five times. Why five times? Isn’t 0 to 5 six numbers? You’re right! But there’s something odd with the range() function. It doesn’t use the last number in the range, it ends there.
Each time the loop runs is called an iteration. In our example, there are five iterations.
You will also see the variable ‘x‘. You can use whatever variable name you want to here.
For each iteration, the variable is set to one of the items that we’ve given the loop. In our example, we’ve given the loop the numbers 0 through to 4. So on the first iteration, ‘x‘ will be ‘0’. On the second iteration, ‘x‘ will be ‘1’.
If we use a list instead of a range of numbers, ‘x‘ would be set to items in the list. We’ll see that soon.
Each loop is called an ‘Iteration’
Within the loop, we have a block of code. It’s a very simple block in our case, we’re only calling the print function. The code is indented under the ‘for … in‘, just like it is for an ‘if … else‘ block. This is how Python knows which code belongs to this loop.
The result of our code is that Python will print the value of ‘x‘ for each loop. The loop will repeat until all numbers in the range have been used.
‘For’ Loops and Lists
Let’s try it again with a list, instead of a range of numbers. Try out this code, and see what happens:
item_list = ['hello', 'world', 'this', 'is', 'my', 'program'] for item in item_list: print (item)
The first thing we’re doing is creating a list of six items. That’s nothing new to you!
Next, we set up the loop. This time, our variable is named ‘item‘, and with each iteration, it will contain an item from ‘item_list‘. It will go through the list in order.
And of course, we have a code block. This is very simply printing each item, one at a time.
A Challenge!
Do you want to try this out?
How would you use a For loop to print each letter in the work ‘Python’?
HINT: Remember that a string behaves like a list.
Solution
We can create this simple code to print each character, one at a time:
for character in 'Python': print (character)
This works the same as if we used a list. Really, strings behave like lists, where each item is a character.