Interrupting a Loop With ‘Break’
When does a loop end? Well, that depends… A For loop will end when it runs out of items to process. A While loop will end when the expression is false.
Sometimes though, we don’t want our loops to run all the way to the end. An example of this is if we’re using a loop to search through some information. Once we’ve found what we’re looking for, we don’t want the loop to keep on running.
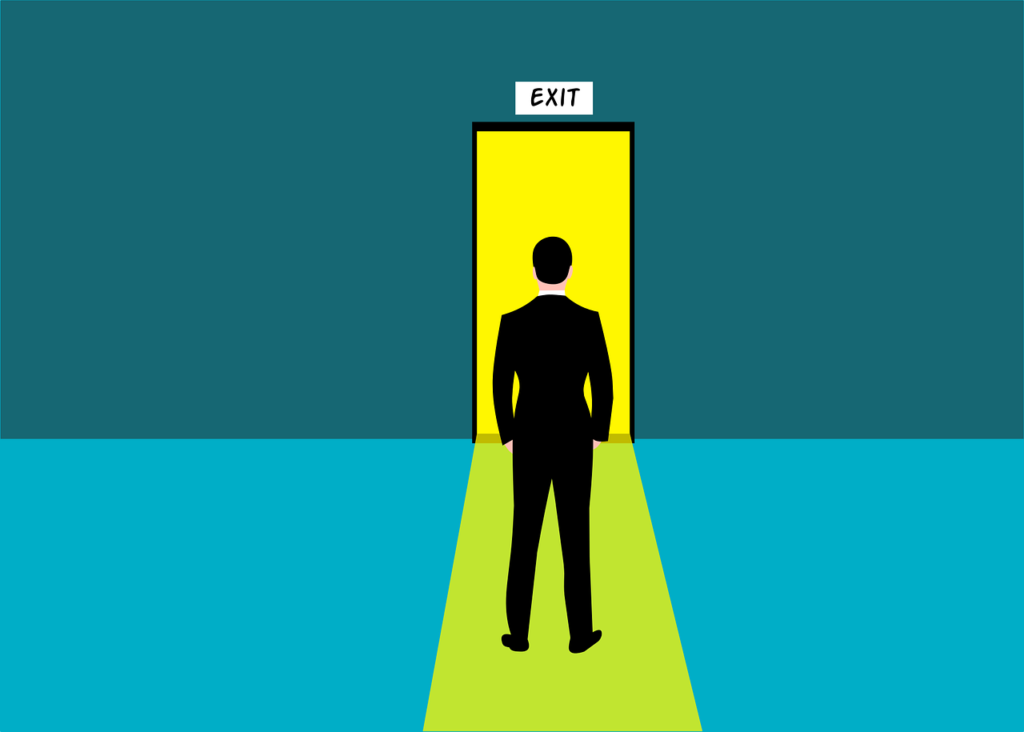
In Python, we do this with the break statement. Try out this example:
sentence = 'Hello World' x = 0 while x < len (sentence): print (sentence[x]) if (sentence[x] == 'W'): break x += 1
This example is very similar to the one we used with While loops. It takes a sentence, and prints each character to screen, one at a time. Except, we’ve added in an if block. Can you see what it does?
The if block looks for the letter ‘W’. If it finds a ‘W’, it will break out of the loop. This means that the ‘For’ or ‘While’ loop will end immediately.
Interrupting a Loop with ‘Continue’
Breaking out of the loop entirely might be a little too extreme in some cases. We have another option called ‘continue‘. This won’t end the entire loop, just the iteration.
Here is an example:
sentence = 'Hello World' x = 0 while x < len (sentence): if (sentence[x] == 'W'): x += 1 continue print (sentence[x]) x += 1
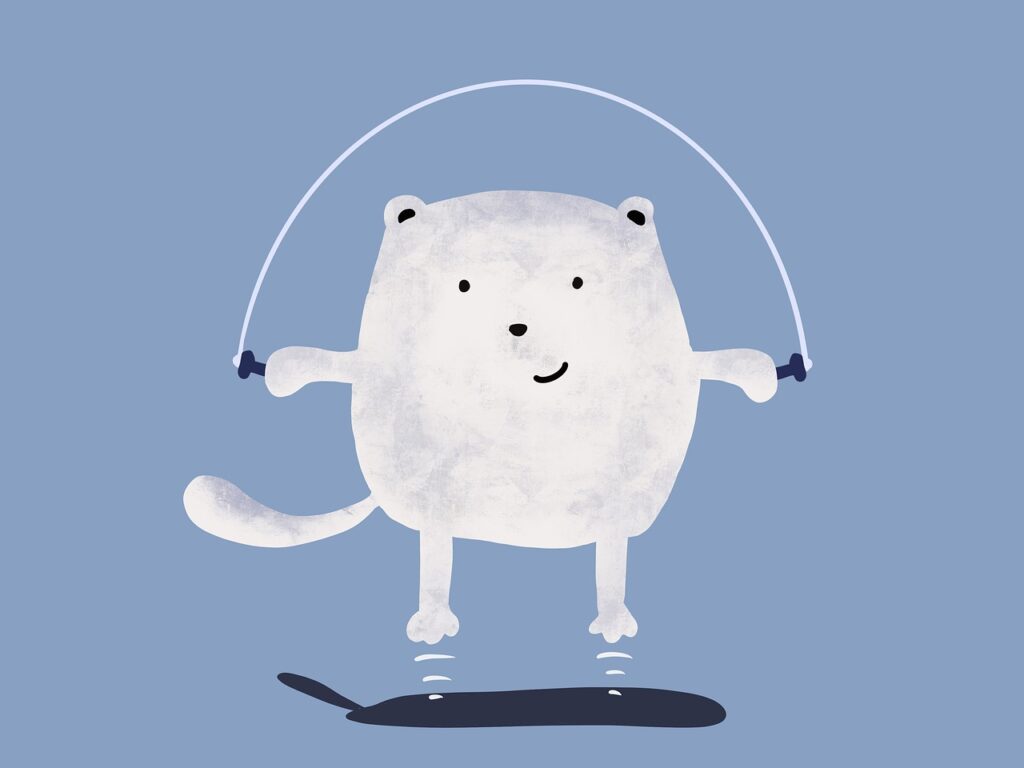
This is very similar to the ‘break’ example. This time, if a ‘W’ character is found, we use ‘continue’, which skips the rest of the iteration. That means ‘W’ won’t be printed, but everything else will.
The order we write the code plays a big part. Notice that in this example, the ‘if’ block comes before print? If it came after, the letter ‘W’ would be printed before the ‘continue’ is run.
Can you also see that we’re updating ‘x’ within the ‘if’ block? Can you see why we would do this? If we don’t do this here, then ‘x’ will never get updated, because we’ll ‘continue’ too early. Try taking it out, and see what happens.