Comparison Operators
When we ask Python questions, it will only answer with yes or no. Well, technically, it will use true or false. This means we need to be specific in the questions we ask.
For example, when people talk to each other, they may say something like “how do you feel today?” This doesn’t work with computers. Remember, they can only answer with true or false (yes or no).
Instead, we might ask “do you feel happy?” See how this can be answered with a yes or no?
To ask a computer questions like these, we compare one thing (perhaps a variable) to another. Something like, “does feelings equal happy?”. The computer could then answer true or false, depending on how it felt that day.
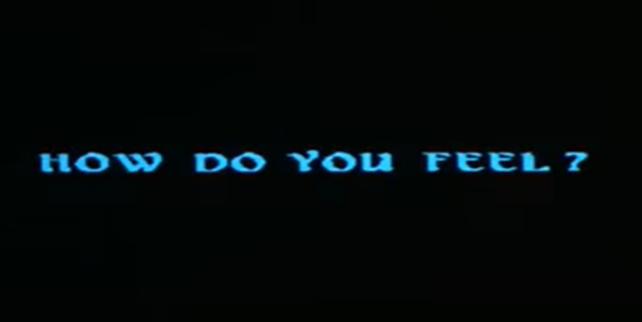
What is a Comparison Operator?
To compare things, we use comparison operators. Think of this example, using the Python shell:
>>> a = 7 >>> b = 9 >>> print (a == b) False
We’ve got two variables, a and b. We’re comparing a and b, and printing the result. a == b is the question we’re asking (does ‘a’ equal ‘b’?). This is called an expression. Notice that the expression has two ‘equals’ symbols?
If we use a single ‘equals’ symbol, we’re telling Python to do something. For example, a = 7 assigns the value of 7 to the variable a. So, a single ‘equals’ is an assignment operator.
If we use two ‘equals’ symbols, we’re asking Python a question. We’re asking “is a equal to b?” We’re comparing the variables, and Python will give us a True or False answer. That makes ‘==‘ a comparison operator.
Remember not to mix them up, or your program will have some funny results!
Comparing Values
Python has six comparison operators:
Comparison | Operator |
---|---|
Equals | == |
Does not equal | != |
Less than | < |
Less than or equal to | <= |
Greater than | > |
Greater than or equal to | >= |
We use all these operators in the same way:
>>> a = 7 >>> b = 9 >>> print (a < b) True
Try a few more out yourself!