Using Python Files
We can either use the Python Shell, or we can save our code in files.
So far, we’ve been using the Python shell and typing in commands. This is good for quickly testing something, but it also comes with a downside. If we have a program we want to run, we need to type in all the commands every time.
What we can do instead, is save our Python code in a file. Then we run the code whenever we want to, without typing anything in. We can also make small changes to our code to make it better.
Let’s try it out. Please open a text editor. Notepad is the default one in Windows, but I prefer either Notepad++ or Atom. If you’re using Linux, you might use nano or vi (or one of the many others).
Please type this code into your text editor:
# Setup variables age = 108 name = 'Joe King' # Print to Screen print ('Hello World, this is my first Python program') print ('my name is ' + name + '. I am', age, 'years old')
Now, save the file. The file name needs to end with .py
For example, you might save your file in Documents (if you’re using Windows) or your home directory (if you’re using Linux), and call it test_code.py
If you’re using Windows, when you save, make sure you change the save as type to All Files.
If you don’t, Notepad will add .txt to the file name.

Explaining the Code
Let’s think about what we’re doing with this code.
The first thing we are doing is setting up two variables called age (an integer) and name (a string).
Feel free to adjust these to your own age and name if you want to.
# Setup variables age = 108 name = 'Joe King'
Then, we print two lines of text to the screen. The second line is the most interesting.
There are a few strings here (like ‘my name is ‘) and some variables. They are being joined together (or concatenated), and then printed to the screen.
# Print to Screen print ('Hello World, this is my first Python program') print ('my name is ' + name + '. I am', age, 'years old')
Concatenation and Lists
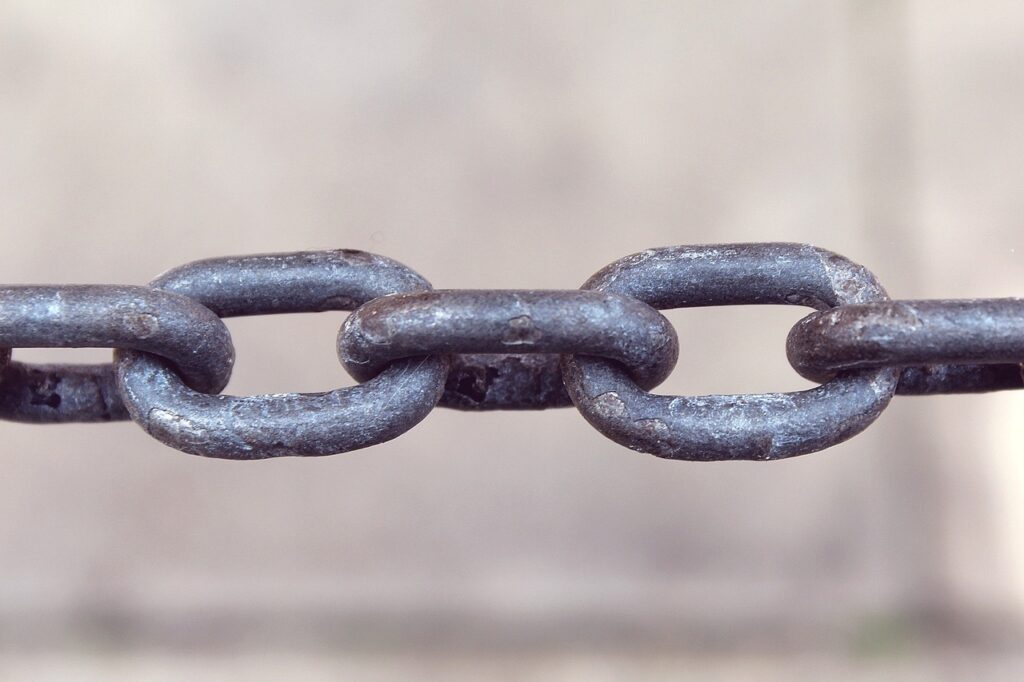
Concatenation
Concatenation is used with strings. This is where we join strings together to make a bigger string
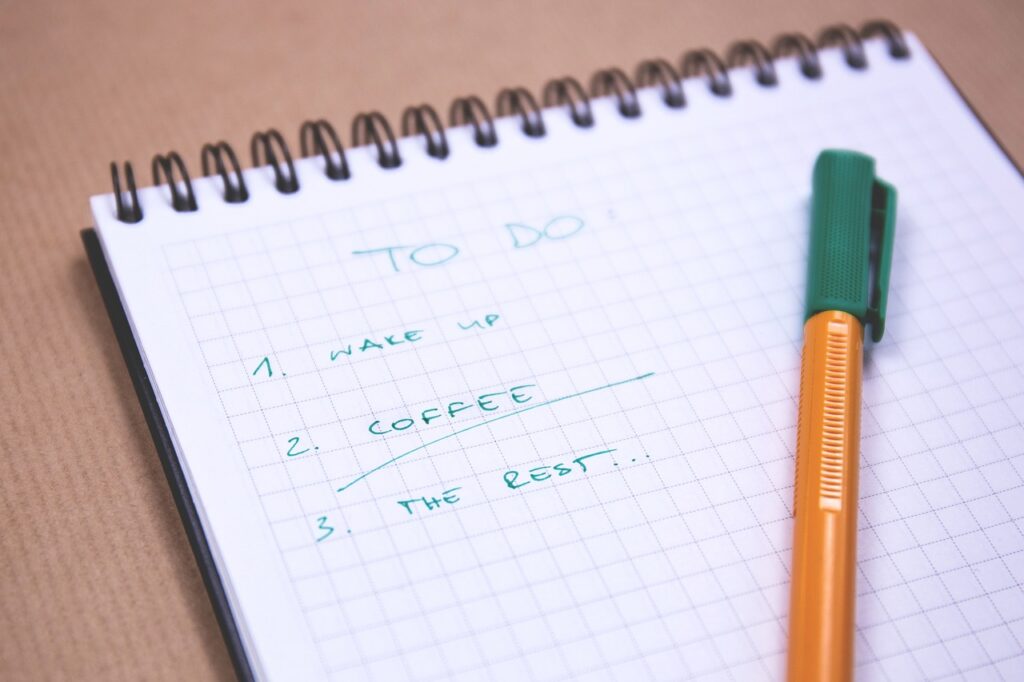
Lists
Lists are a new data type. They are like a shopping list or a to-do list, containing items.
Comments
I’m sure you’ve also noticed something new in this code. We’ve added lines starting with a hash symbol, like these:
# Setup variables
In Python, any line that starts with a hash symbol is a comment. This is a line of text that Python ignores completely.
What’s the point if Python ignores comments completely? They are there for us, the programmers. They allow us to leave notes on what our code is doing.
This helps us if we come back to our code after months, and we don’t quite remember what it’s doing (yeah, really, it’s easy to forget what code does if you haven’t seen it in a while).
Comments also help other programmers to understand what we’re doing with our code (if we decide to share it).
Running the Code
That’s enough explaining, it’s now time to run our code!
To do this, open a command prompt (Windows) or terminal (Linux). Then move into the directory where you saved your program (such as documents or home).
From this location, you can run python test_code.py (on Windows) or python3 test_code.py
That’s how you run Python programs!
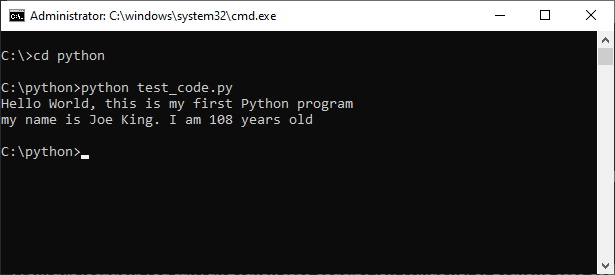
If you want something to try out, see what happens if you double click your python file.
Integrated Development Environment: Using IDLE
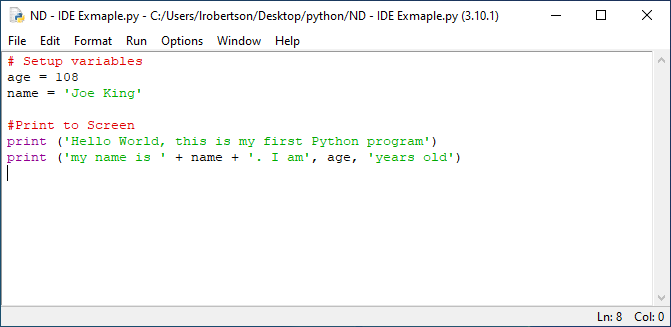
Now that we’re using more than just the shell, it’s a good time to introduce IDLE, and see how to use it. It makes development (writing programs) much easier for us.
Next…
Are you ready to practice the skills you’ve learned so far? Next, you’re going to use Python to solve some simple challenges.