Fun With Lists
There are a few nice things we can do with lists. We’ll take a look at just a few of them here. Please open a Python shell, and follow along.
Length
We can use the len() function to find the length of a list. This means, how many items are in the list.
mylist = [12, 48, 6, 108, 37] len (mylist) # Function
In this example, we have a list with five items. When we use len() it returns a length of 5.
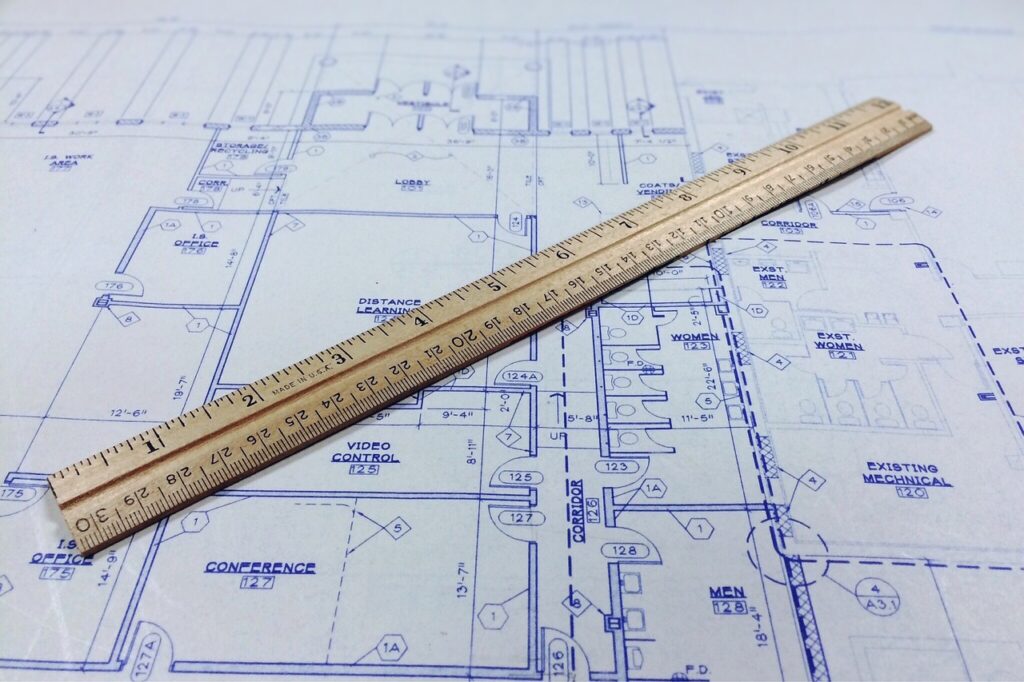
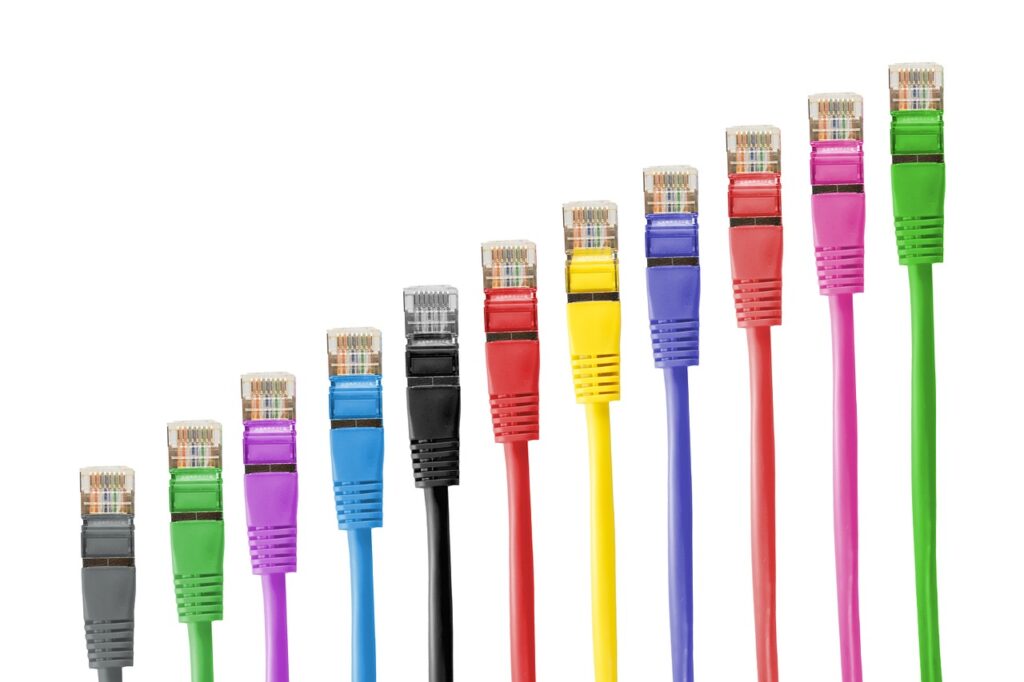
Sorting Items in Lists
Sometimes we will want to put the items in a list in order. For this, we can use the sort method. A method is very much like a function, but it ‘belongs’ to an object.
In this example, the sort() method belongs to the mylist object. You can see how this looks like a function but is a little different.
mylist = [12, 48, 6, 108, 37] # This updates the original list, not just prints the list in order mylist.sort() # Method print (mylist)
When we use the sort function, the items in the list aren’t printed on the screen. Instead, the method sorts the items within the list. If we then print the list, we can see that the items are in order.
Adding Items to Lists
We can add, or append, new items to a list with the append method.
mylist = [12, 48, 6, 108, 37] mylist.append (14) print (mylist)
In this example, ’14’ is added as a new item in the list. There are already five items in the list, so this becomes the sixth item.
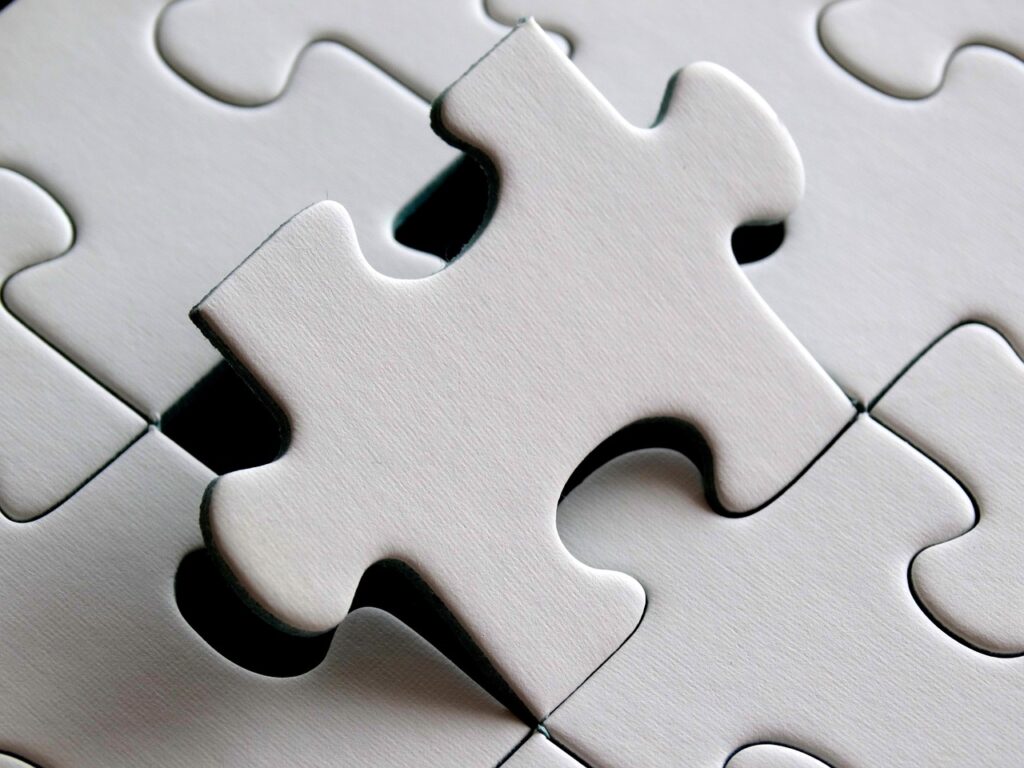
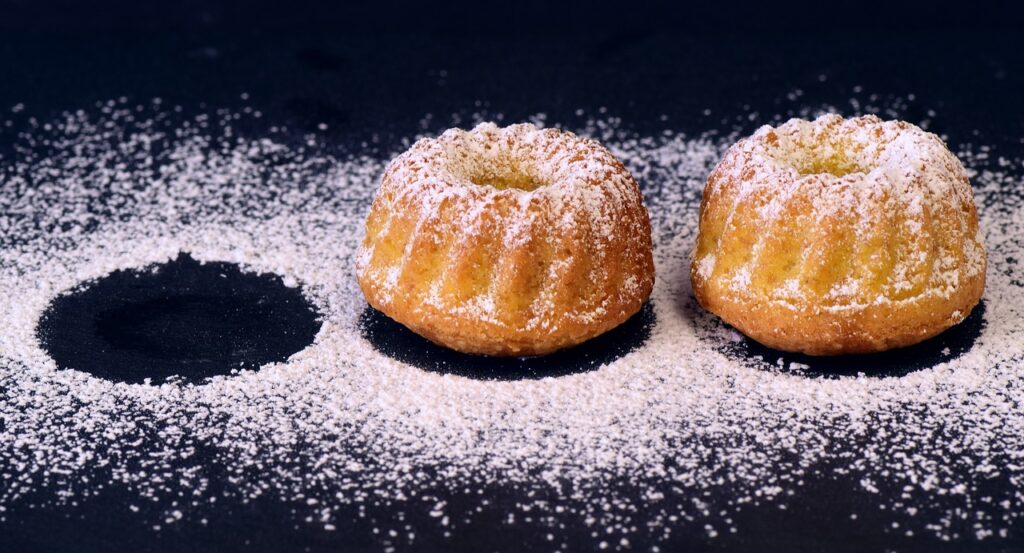
Removing Items from Lists
Building on the last example, we can also remove items from the end of the list with the pop method. This method prints the last item in the list to the screen, and then removes it from the list.
mylist.pop() print (mylist)
Inserting Items
And for our final example, we can add items to the list with the insert method. This is different than the append method, which always puts the new item at the end of the list.
mylist.insert (1, 5) '5' is added to the list as element #1 print (mylist)
In this example, we created a new item with the value ‘5’. We inserted it into the list as element number 1. Keep in mind that computers count from zero, so element #1 is the second item in the list.
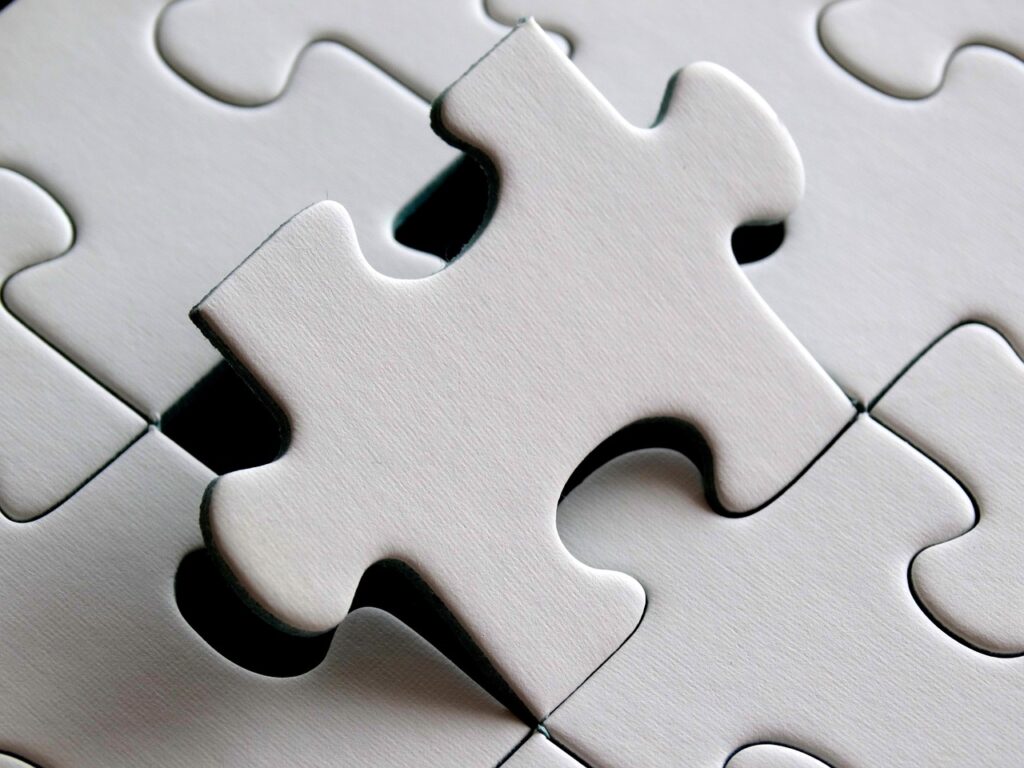