A List of Lists
Lists can contain pretty much anything. Integers, strings, booleans, whatever really. This even includes other lists! We can call these nested lists.
Imagine you’re going shopping, and have three shops you want to go to. You might have a list for dairy, the butcher, and the baker (sorry vegans!)
You could have three separate lists, or you could have a master list containing them all.
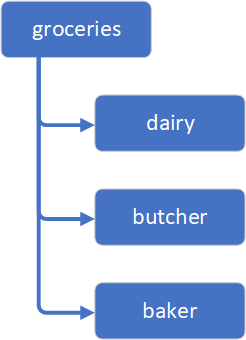
Creating Nested Lists
Let’s see how this might work in Python. Please open your Python shell, and follow along.
First, we’ll create our dairy, butcher, and baker lists:
>>> dairy = ['eggs', 'milk', 'cheese' ] >>> butcher = ['sausages', 'bacon'] >>> baker = ['bread', 'rolls', 'muffins']
Next, we can create our master list, called groceries. Each item in this list is one of our other lists:
>>> groceries = [dairy, butcher, baker]
When we print the groceries list to the screen, we can see the lists which are nested within.
>>> groceries [['eggs', 'milk', 'cheese'], ['sausages', 'bacon'], ['bread', 'rolls', 'muffins']]
Of course, we can still look at items with each list. We can even look at items in the ‘child’ lists, by telling Python about more elements. We can even get individual characters from a string if we want to.
# Prints ['sausages', 'bacon'] print (groceries [1]) # Prints 'sausages' print (groceries [1][0]) # Prints 'a' print (groceries [1][0][1])